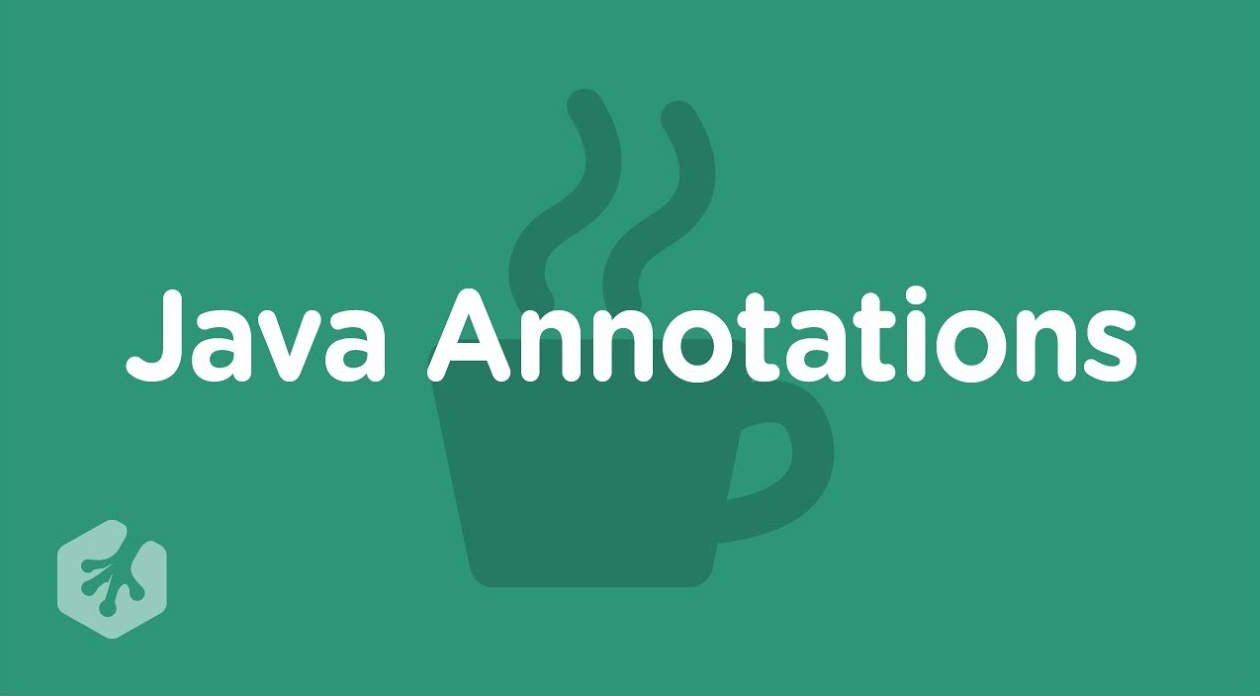
1. Introduction to Annotations in Java
Annotations in Java are metadata that provide additional information about a program element, such as a class, method, or field. Annotations are used to provide information to the compiler or to other tools, such as code generators or libraries, and can be used for a variety of purposes, including defining access control, providing information about dependencies, or specifying runtime behavior.
In Java, annotations are created using the @interface
keyword and can be
applied to any program element that is annotated with the @Target
meta-annotation. Some of the commonly used annotations in Java include
@Override
, @Deprecated
, and @SuppressWarnings
.
2. Creating a Custom Annotation in Java
To create a custom annotation in Java, follow these steps:
- Create a new Java interface and name it appropriately, starting with the
@
symbol. - Add the
@interface
keyword before the name of the interface to indicate that it is an annotation. - Add any required attributes to the annotation using methods with no parameters and no return value.
- Add the
@Retention
meta-annotation to indicate the level of retention for the annotation, such as RetentionPolicy.RUNTIME to retain the annotation at runtime or RetentionPolicy.SOURCE to retain the annotation only in the source code.
For example, consider the following custom annotation:
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Retention(RetentionPolicy.RUNTIME)
public @interface Author {
String name();
int year();
}
This annotation provides information about the author of a class, method, or field and includes two attributes, name and year.
3. Using Custom Annotations in Java
To use a custom annotation in Java, simply apply the annotation to the desired
program element, such as a class, method, or field. The following example
demonstrates how to use the @Author
annotation:
@Author(name = "John Doe", year = 2020)
public class MyClass {
// ...
}
4. Accessing Custom Annotations at Runtime
To access custom annotations at runtime, you can use the java.lang.reflect
package, which provides classes for working with annotations and reflection. For
example, the following code demonstrates how to access the @Author
annotation
for a given class:
Class<?> clazz = MyClass.class;
Author author = clazz.getAnnotation(Author.class);
System.out.println("Author: " + author.name());
System.out.println("Year: " + author.year());
5. Conclusion
In conclusion, custom annotations in Java are a powerful tool for adding metadata to your code, providing additional information about program elements, and enabling new functionality in tools and libraries. By creating and using your own custom annotations, you can streamline your development process and make your code more organized and maintainable.